20 Jan 2018
#JavaScript
JavaScript is easy to learn. :))
JavaScript Data Types
Primitive Data and Complex Data types
• null
• undefined
• boolean
• number
• string
• object
JavaScript Types are Dynamic.
var x; // Now x is undefined
var x = 5; // Now x is a Number
var x = "John"; // Now x is a String
Typeof
You can use the JavaScript typeof operator to find the type of a JavaScript variable.
typeof {name:'John', age:34} // Returns "object"
typeof [1,2,3,4] // Returns "object" (not "array", see note below)
typeof null // Returns "object"
typeof function myFunc(){} // Returns "function"
typeof null === "object"; // true
typeof [1,2,3] === "object"; // true
Difference Between Undefined and Null
Undefined and null are equal in value but different in type:
typeof undefined // undefined
typeof null // object
null === undefined // false
null == undefined // true
Function are objects
typeof function a(){ /* .. */ } === "function"; // true
function a(b,c) {
/* .. */
}
a.length; // 2
undefined Versus “undeclared”
Many developers will assume “undefined” and “undeclared” are
roughly the same thing, but in JavaScript, they’re quite different.
undefined is a value that a declared variable can hold. “Undeclared”
means a variable has never been declared.
var a;
a; // undefined
b; // ReferenceError: b is not defined
if (typeof DEBUG !== "undefined") {
console.log( "Debugging is starting" );
}
• null is an empty value.
• undefined is a missing value.
Or:
• undefined hasn’t had a value yet.
• null had a value and doesn’t anymore.
Array
var a = [ 1, "2", [3] ];
a.length; // 3
a[0] === 1; // true
a[2][0] === 3;// true
a[19] = 19;
console.log(a);
Using delete on an array value will remove
that slot from the array , but even if you remove
the final element, it does not update the length
property,
var a = [ ];
a["13"] = 42;
a.length; // 14
a["test"] = "test";
a.test
var a = "foo"
var c = Array.prototype.join.call(a, "-")
Array.prototype.reverse.call(c) // error
c.split("-").reverse().join("-") // split converts string to array
Arithmetic
0.1 + 0.2 === 0.3; // false
0.3 . It’s really close, 0.30000000000000004
“integer” is just a value that has no fractional decimal
value. That is, 42.0 is as much an “integer” as 42 .
NaN
NaN literally stands for “not a number,” though this label/description
is very poor and misleading, as we’ll see shortly. It would be much
more accurate to think of NaN as being an “invalid number,” “failed number,” or even “bad number,” than to think of it as “not a num‐
ber.”
For example:
var a = 2 / "foo"; // NaN
typeof a === "number"; // true
In JavaScript, there are no pointers, and references work a bit differ‐
ently. You cannot have a reference from one JS variable to another
variable
var a;
var b;
b++;
a = 2; //
b = a; // `b` is always a copy of the value in `a`
var c = [1,2,3];
var d = c; // `d` is a reference to the shared `[1,2,3]` value
d.push( 4 );
c; // [1,2,3,4]
d; // [1,2,3,4]
Simple values (aka scalar primitives) are always assigned/passed by
value-copy: null , undefined , string , number , boolean , and ES6’s
symbol .
Compound values objects and functions always create a copy of
the reference on assignment or passing.
var a = [1,2,3];
var b = a;
a; // [1,2,3]
b; // [1,2,3]
// later
b = [4,5,6];
a; // [1,2,3]
b; // [4,5,6]
function foo(x) {
x.push( 4 );
x; // [1,2,3,4]
// later
x.length = 0; // empty existing array in-place
x.push( 4, 5, 6, 7 );
x; // [4,5,6,7]
}
var a = [1,2,3];
foo( a );
a; // [4,5,6,7] not [1,2,3,4]
function foo(x) {
x = x + 1; // 3
}
var a = 2;
var b = new Number( a ); // or equivalently `Object(a)`
foo( b );
console.log( b ); // 2, not 3
The problem is that the underlying scalar primitive value is not
mutable (same goes for String and Boolean). If a Number object
holds the scalar primitive value 2, that exact Number object can never
be changed to hold another value; you can only create a whole new
Number object with a different value.
String
var a = new String( "abc" );
typeof a; // "object" ... not "String"
a instanceof String;
Internal [[Class]]
Object.prototype.toString.call( [1,2,3] );
// "[object Array]"
Object.prototype.toString.call( /regex-literal/i );
// "[object RegExp]"
So, for the array in this example, the internal [[Class]] value is
"Array", and for the regular expression, it’s "RegExp"
Boxing
var a = "abc";
a.length; // 3
a.toUpperCase(); // "ABC"
var a = new String( "abc" );
var b = new Number( 42 );
var c = new Boolean( true );
a.valueOf(); // "abc"
b.valueOf(); // 42
c.valueOf(); // true
var a = new Boolean( false );
if (!a) {
console.log( "Oops" ); // never runs
}
Natives as Constructors
var a = new Array( 1, 2, 3 );
a; // [1, 2, 3]
var b = [1, 2, 3];
b; // [1, 2, 3]
The Array(..) constructor does not require the
new keyword in front of it.
var a = new Array( 3 );
var b = [ undefined, undefined, undefined ];
var c = [];
c.length = 3;
var c = [4,5,6,8];
c.length = 2;
c // [4,5]
var c = new Object();
c.foo = "bar";
c; // { foo: "bar" }
var d = { foo: "bar" };
d; // { foo: "bar" }
var e = new Function( "a", "return a * 2;" );
var f = function(a) { return a * 2; }
function g(a) { return a * 2; }
var h = new RegExp( "^a*b+", "g" );
var i = /^a*b+/g;
Scope
{
}
javascript only function scope
var name = "Jhon";
if(name == "Jhon") {
var surname = "Doe"
}
console.log(name);
console.log(surname);
var name = "Jhon";
function foo() {
if(name == "Jhon") {
var surname = "Doe";
}
}
foo();
console.log(surname) // error
var a = 40;
function myFunc()
var b = 1;
console.log(b);
c = 50;
console.log(c);
}
myFunc();
console.log(c);
let && var && const
var
for(var i = 0; i<10; i++) {
console.log(i)
}
console.log(i)
function(){
for(var i = 0; i<10; i++) {
console.log(i)
}
console.log(i)
}
function printing(){
for(var i = 0; i<10; i++) {
console.log(i)
}
}
printing()
console.log(i)
(function (){
for(var i = 0; i<10; i++) {
console.log(i)
}
})()
let
The let statement declares a block scope local variable
for(let i = 0; i<10; i++) {
console.log(i)
}
console.log(i)
const
Constants are block-scoped, much like variables defined using the let statement. The value of a constant cannot change through re-assignment, and it can’t be redeclared.
const foo = 5;
foo = 10 // this will throw an error
const dog={
age: 3
}
dog.age = 5
dog = { name: 'biko'}
Object
var user = {
name: "Jhon",
say: function (name) { console.log(name); }
};
var Person = function (name) {
this.name = name;
};
Person.prototype.say = function (words) {
alert(this.name + ' says "' + words + '"');
};
var jhon = new Person('Jhon');
console.log(jhon);
jhon.say("Hi");
function User(name) {
// this = {}; (implicitly)
// add properties to this
this.name = name;
this.isAdmin = false;
// return this; (implicitly)
}
var user = new User("Jhon");
let user = {
name: "John",
age: 30,
isAdmin: true
};
for(let key in user) {
// keys
alert( key ); // name, age, isAdmin
// values for the keys
alert( user[key] ); // John, 30, true
}
var myObject = {};
Object.defineProperty( myObject, "a", {
value: 2,
writable: true,
configurable: true,
enumerable: true
} );
myObject.a; // 2
var myObject = {};
Object.defineProperty( myObject, "a", {
value: 2,
writable: false, // not writable!
configurable: true,
enumerable: true
} );
myObject.a = 3;
myObject.a; // 2
var myObject = {
a: 2
};
myObject.a = 3;
myObject.a; // 3
Object.defineProperty( myObject, "a", {
value: 4,
writable: true,
configurable: false, // not configurable!
enumerable: true
} );
myObject.a; // 4
myObject.a = 5;
myObject.a; // 5
Object.defineProperty( myObject, "a", {
value: 6,
writable: true,
configurable: true,
enumerable: true
} ); // TypeError
var myObject = {
a: 2
};
myObject.a; // 2
Global object
When JavaScript was created, there was an idea of a “global object” that provides all global variables and functions
References
11 Nov 2017
In Java, all classes inherit from Object, but there is no such generic super-class in PHP.
Major 3 things I always remember for OO PHP does not have:
- PHP has no main function for classes.
- Like C++ you have declare a constructor and destructor i.e. __construct()
- You cannot declare final (constant) to your variables, but to methods and classes so that they cannot be overrided and inherited repsectivley.
The include (or require) statement takes all the text/code/markup that exists in the specified file and copies it into the file that uses the include statement.
The include and require statements are identical, except upon failure:
require will produce a fatal error (E_COMPILE_ERROR) and stop the script
include will only produce a warning (E_WARNING) and the script will continue
include('pageBanner.php');
or
require('pageBanner.php');
Assume we have a standard footer file called “footer.php”, that looks like this:
// footer.php
<?php
echo "<p>Copyright © 1999-" . date("Y") . " W3Schools.com</p>";
?>
<!-- index.html -->
<html>
<body>
<h1>Welcome to my home page!</h1>
<p>Some text.</p>
<?php include 'footer.php';?>
</body>
</html>
Autoloading
PHP doesn’t have packages, but it does have namespaces.
namespace B
{
class A
{
public static function say()
{
echo 'Я пространство имен B';
}
}
}
namespace A;
class A
{
public static function say()
{
echo 'Я пространство имен А';
}
}
require_once 'A.php';
require_once 'B.php';
use A\A;
use B\A
A\A::say();
B\A::say();
Autoloading allows us to use PHP classes without the need to require() or include()
__autoload — Attempt to load undefined class
<?php
use App\Controller\MyClass as MC;
$obj = new MC();
echo $obj->WhoAmI();
function __autoload($class) {
$class = 'classes\' . str_replace('\\', '/', $class) . '.php';
require_once($class);
}
spl_autoload_register — Register given function as __autoload() implementation
<?php
class Autoloader {
static public function loader($className) {
$filename = "Classes/" . str_replace("\\", '/', $className) . ".php";
if (file_exists($filename)) {
include($filename);
if (class_exists($className)) {
return TRUE;
}
}
return FALSE;
}
}
spl_autoload_register('Autoloader::loader');
Composer
Dependency Manager for PHP
Composer solves the following problems:
- dependency resolution for PHP packages
- autoloading solution for PHP packages
- keeping all packages updated
Create and empy file in the myproject folder, called composer.json:
/var/www/myproject/composer.json
Kint - debugging helper for PHP developers
{
"require": {
"raveren/kint": "0.9"
}
}
composer install
Composer has created a folder named vendor in your project
//index.php
<?php
require 'vendor/autoload.php';
This loads the Composer autoloader. So this line includes everything that Composer has downloaded into the project.
// create demo data
$variable = [1, 17, "hello", null, [1, 2, 3]];
// use KINT directly (which has been loaded automatically via Composer)
d($variable);
{
"require": {
"raveren/kint": "0.9",
"phpmailer/phpmailer": "5.2.*"
}
}
Dependency Injection
More specifically, dependency injection is effective in these situations:
You need to inject configuration data into one or more components.
You need to inject the same dependency into multiple components.
You need to inject different implementations of the same dependency.
You need to inject the same implementation in different configurations.
You need some of the services provided by the container.
Dependency injection is not effective if:
You will never need a different implementation.
You will never need a different configuration.
Advantages of DI
Reduces class coupling
Increases code reusability
Improves code maintainability
Improves application testing
Centralized configuration
Drawback
The main drawback of dependency injection is that using many instances together can become a very difficult if there are too many instances and many dependencies that need to be resolved.
There are at least three ways an object can receive a reference to an external module
- constructor injection: the dependencies are provided through a class constructor.
- setter injection: the client exposes a setter method that the injector uses to inject the dependency.
- interface injection: the dependency provides an injector method that will inject the dependency into any client passed to it. Clients must implement an interface that exposes a setter method that accepts the dependency.
Constructor injection
This method requires the client to provide a parameter in a constructor for the dependency.
// Constructor
Client(Service service) {
// Save the reference to the passed-in service inside this client
this.service = service;
}
Setter injection
This method requires the client to provide a setter method for the dependency.
// Setter method
public void setService(Service service) {
// Save the reference to the passed-in service inside this client
this.service = service;
}
Interface injection
This is simply the client publishing a role interface to the setter methods of the client’s dependencies. It can be used to establish how the injector should talk to the client when injecting dependencies.
// Service setter interface.
public interface ServiceSetter {
public void setService(Service service);
}
public class Client implements ServiceSetter {
// Internal reference to the service used by this client.
private Service service;
// Set the service that this client is to use.
@Override
public void setService(Service service) {
this.service = service;
}
}
Magento
Magento is the World’s #1 Commerce Platform
Magento is the leading platform for open commerce innovation. Every year, Magento handles over $100 billion in gross merchandise volume
Magento is the leading eCommerce platform used for online stores. It is a high-performant, scalable solution with powerful out of the box functionality and a large community built around it that continues to add new features.
CE AND EE
- MAGENTO - (2008)
- MAGENTO 2 - (2015)
Magento2 Object Manager
Magento uses constructor injection to provide dependencies through an object’s class constructor.
Overview
Large applications, such as the Magento application, use an object manager to avoid boilerplate code when composing objects during instantiation.
Responsibilities
The object manager has the following responsibilities:
Object creation in factories and proxies.
Implementing the singleton pattern by returning the same shared instance of a class when requested.
Dependency management by instantiating the preferred class when a constructor requests its interface.
Automatically instantiating parameters in class constructors.
Configuration
The di.xml file configures the object manager and tells it how to handle dependency injection.
This file specifies the preferred implementation class the object manager generates for the interface declared in a
class constructor.
The di.xml file
Overview
The di.xml file configures which dependencies to inject by the object manager.
Areas and application entry points
Each module can have a global and area-specific di.xml file. Magento reads all the di.xml configuration files declared in the system and merges them all together by appending all nodes.
As a general rule, the area specific di.xml files should configure dependencies for the presentation layer, and your module’s global di.xml file should configure the remaining dependencies.
Magento loads The configuration in the following stages:
- Initial (app/etc/di.xml)
- Global (/etc/di.xml)
- Area-specific (/etc//di.xml)
Examples:
- In index.php, the \Magento\Framework\App\Http class loads the area based on the front-name provided in url.
- In static.php, the \Magento\Framework\App\StaticResource class also loads the area based on the url in the request.
- In cron.php, the \Magento\Framework\App\Cron class always loads the ‘crontab’ area.
Type configuration
You can configure the type in your di.xml configuration node in the following ways:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<virtualType name="moduleConfig" type="Magento\Core\Model\Config">
<arguments>
<argument name="type" xsi:type="string">system</argument>
</arguments>
</virtualType>
<type name="Magento\Core\Model\App">
<arguments>
<argument name="config" xsi:type="object">moduleConfig</argument>
</arguments>
</type>
</config>
The preceding example declares the following types:
- moduleConfig: A virtual type that extends the type Magento\Core\Model\Config.
- Magento\Core\Model\App: All instances of this type receive an instance of moduleConfig as a dependency.
Virtual types
A virtual type allows you to change the arguments of a specific injectable dependency and change the behavior of a particular class. This allows you to use a customized class without affecting other classes that have a dependency on the original.
The example creates a virtual type for Magento\Core\Model\Config and specifies system as the constructor argument for type.
Constructor arguments
You can configure the class constructor arguments in your di.xml in the argument node. The object manager injects these arguments into the class during creation. The name of the argument configured in the XML file must correspond to the name of the parameter in the constructor in the configured class.
The following example creates instances of Magento\Core\Model\Session with the class constructor argument $sessionName set to a value of adminhtml:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Core\Model\Session">
<arguments>
<argument name="sessionName" xsi:type="string">adminhtml</argument>
</arguments>
</type>
</config>
Abstraction-implementation mappings
The object managers uses the abstraction-implementation mappings when the constructor signature of a class requests an object by its interface. The object manager uses these mappings to determine what the default implementation is for that class for a particular scope.
The preference node specifies the default implementation:
<!-- File: app/etc/di.xml -->
<config>
<preference for="Magento\Core\Model\UrlInterface" type="Magento\Core\Model\Url" />
</config>
This mapping is in app/etc/di.xml, so the object manager injects the Magento\Core\Model\Url implementation class wherever there is a request for the Magento\Core\Model\UrlInterface in the global scope.
<!-- File: app/code/core/Magento/Backend/etc/adminhtml/di.xml -->
<config>
<preference for="Magento\Core\Model\UrlInterface" type="Magento\Backend\Model\Url" />
</config>
This mapping is in app/code/core/Magento/Backend/etc/adminhtml/di.xml, so the object manager injects the Magento\Backend\Model\Url implementation class wherever there is a request for the Magento\Core\Model\UrlInterface in the admin area.
Parameter configuration inheritance
Parameters configured for a class type pass on its configuration to its descendant classes. Any descendant can override the parameters configured for its supertype; that is, the parent class or interface:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Framework\View\Element\Context">
<arguments>
<argument name="urlBuilder" xsi:type="object">Magento\Core\Model\Url</argument>
</arguments>
</type>
<type name="Magento\Backend\Block\Context">
<arguments>
<argument name="urlBuilder" xsi:type="object">Magento\Backend\Model\Url</argument>
</arguments>
</type>
</config>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Filesystem" shared="false">
<arguments>
<argument name="adapter" xsi:type="object" shared="false">Magento\Filesystem\Adapter\Local</argument>
</arguments>
</type>
</config>
Injection types used in Magento
This section explains the two dependency injection types used in Magento using the following example:
namespace Magento\Backend\Model\Menu;
class Builder
{
/**
* @param \Magento\Backend\Model\Menu\Item\Factory $menuItemFactory
* @param \Magento\Backend\Model\Menu $menu
*/
public function __construct(
Magento\Backend\Model\Menu\Item\Factory $menuItemFactory, // Service dependency
Magento\Backend\Model\Menu $menu // Service dependency
) {
$this->_itemFactory = $menuItemFactory;
$this->_menu = $menu;
}
public function processCommand(\Magento\Backend\Model\Menu\Builder\CommandAbstract $command) // API param
{
// processCommand Code
}
}
Let’s examine how this works on a simple example:
class A
{
public function __construct(B $b)
{
$this->_b = $b;
}
}
When creating object from class A, the following would happen:
- ObjectManager->create(‘A’) is called
- Constructor of A is examined
- Class B is being created, and used for creating A
Magento 2, they are separated into two groups: injectable, and non-injectable.
Injectable: Service objects that are singletons obtained through dependency injection. The object manager uses the configuration in the di.xml file to create these objects and inject them into constructors.
Newable/non-injectable: Objects obtained by creating a new class instance every time. Transient objects, such as those that require external input from the user or database, fall into this category. Attempts to inject these objects produce either an error that the object could not be created or an incorrect object that is incomplete.
Factories
Factories are service classes that instantiate non-injectable classes, that is, models that represent a database entity
The following example illustrates the relationship between a simple factory and the ObjectManager:
namespace Magento\Framework\App\Config;
class BaseFactory
{
/**
* @var \Magento\Framework\ObjectManagerInterface
*/
private $_objectManager;
/**
* @param \Magento\Framework\ObjectManagerInterface $objectManager
*/
public function __construct(\Magento\Framework\ObjectManagerInterface $objectManager)
{
$this->_objectManager = $objectManager;
}
/**
* Create config model
* @param string|\Magento\Framework\Simplexml\Element $sourceData
* @return \Magento\Framework\App\Config\Base
*/
public function create($sourceData = null)
{
return $this->_objectManager->create(\Magento\Framework\App\Config\Base::class, ['sourceData' => $sourceData]);
}
}
Using factories
You can get the singleton instance of a factory for a specific model using dependency injection.
The following example shows a class getting the BlockFactory instance through the constructor:
function __construct ( \Magento\Cms\Model\BlockFactory $blockFactory) {
$this->blockFactory = $blockFactory;
}
Calling the create() method on a factory gives you an instance of its specific class:
$block = $this->blockFactory->create();
// or
$resultItem = $this->itemFactory->create([
'title' => $item->getQueryText(),
'num_results' => $item->getNumResults(),
]);
Proxies
Magento’s constructor injection pattern enables you to flexibly manage your class dependencies.
If a class’s constructor is particularly resource-intensive, this can lead to unnecessary performance impact when another class depends on it
As an example, consider the following two classes:
class SlowLoading
{
public function __construct()
{
// ... Do something resource intensive
}
public function getValue()
{
return 'SlowLoading value';
}
}
class FastLoading
{
protected $slowLoading;
public function __construct(
SlowLoading $slowLoading
){
$this->slowLoading = slowLoading;
}
public function getFastValue()
{
return 'FastLoading value';
}
public function getSlowValue()
{
return $this->slowLoading->getValue();
}
}
Proxies are generated code
Magento has a solution for this situation: proxies. Proxies extend other classes to become lazy-loaded versions of them. That is, a real instance of the class a proxy extends created only after one of the class’s methods is actually called.
Using the preceding example, a proxy can be passed into the constructor arguments instead of the original class, using DI configuration as follows:
<type name="FastLoading">
<arguments>
<argument name="slowLoading" xsi:type="object">SlowLoading\Proxy</argument>
</arguments>
</type>
With the proxy used in place of SlowLoading, the SlowLoading class will not be instantiated—and therefore, the resource intensive constructor operations not performed—until the SlowLoading object is used (that is, if the getSlowValue method is called).
References
29 Mar 2017
Writing correct programs is hard; writing correct concurrent programs is harder.
Multitasking
Process
In computing, a process is an instance of a computer program that is being executed. It contains the program code and its current activity. Depending on the operating system (OS), a process may be made up of multiple threads of execution that execute instructions concurrently.
Process memory is divided into four sections for efficient working :
- The Text section is made up of the compiled program code, read in from non-volatile storage when the program is launched.
- The Stack contains the temporary data such as method/function parameters, return address and local variables.
- The Heap is used for the dynamic memory allocation during its run time.
- The Data section is made up the global and static variables, allocated and initialized prior to executing the main.
Process States
When a process executes, it passes through different states.
Processes in the operating system can be in any of the following states:
NEW(Start)- The process is being created.
READY- The process is waiting to be assigned to a processor.
RUNNING- Instructions are being executed.
WAITING- The process is waiting for some event to occur(such as an I/O completion or reception of a signal).
TERMINATED(Exit)- The process has finished execution.
Process Control Block
A Process Control Block is a data structure maintained by the Operating System for every process.
The PCB is identified by an integer process ID (PID).
S.N |
Information |
Description |
1 |
Process State |
The current state of the process i.e., whether it is ready, running, waiting, or whatever. |
2 |
Process privileges |
This is required to allow/disallow access to system resources. |
3 |
Process ID |
Unique identification for each of the process in the operating system. |
4 |
Pointer |
A pointer to parent process. |
5 |
Program Counter |
Program Counter is a pointer to the address of the next instruction to be executed for this process. |
6 |
CPU registers |
Various CPU registers where process need to be stored for execution for running state. |
7 |
CPU Scheduling Information |
Process priority and other scheduling information which is required to schedule the process. |
8 |
Memory management information |
This includes the information of page table, memory limits, Segment table depending on memory used by the operating system. |
9 |
Accounting information |
This includes the amount of CPU used for process execution, time limits, execution ID etc. |
10 |
IO status information |
This includes a list of I/O devices allocated to the process. |
Process Scheduling
The process scheduling is the activity of the process manager that handles the removal of the running process from the CPU and the selection of another process on the basis of a particular strategy.
Process scheduling is an essential part of a Multiprogramming operating systems.
Process Scheduling Queues
The OS maintains all PCBs in Process Scheduling Queues. The OS maintains a separate queue for each of the process states and PCBs of all processes in the same execution state are placed in the same queue. When the state of a process is changed, its PCB is unlinked from its current queue and moved to its new state queue.
The Operating System maintains the following important process scheduling queues −
- Job queue − This queue keeps all the processes in the system.
- Ready queue − This queue keeps a set of all processes residing in main memory, ready and waiting to execute. A new process is always put in this queue.
- Device queues − The processes which are blocked due to unavailability of an I/O device constitute this queue.
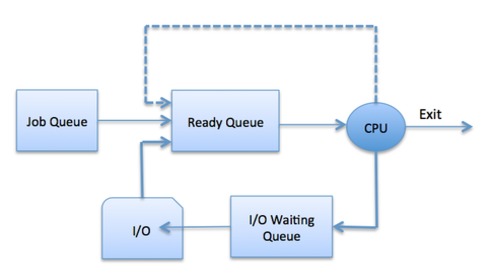
Types of Schedulers
- Long Term Scheduler
- Medium Term Scheduler
- Short Term Scheduler
Context switch
The process of a Context Switch
In computing, a context switch is the process of storing the state of a process or of a thread, so that it can be restored and execution resumed from the same point later. This allows multiple processes to share a single CPU, and is an essential feature of a multitasking operating system
Some operating systems also require a context switch to move between user mode and kernel mode tasks. The process of context switching can have a negative impact on system performance, although the size of this effect depends on the nature of the switch being performed.
When the process is switched, the following information is stored for later use.
- Program Counter
- Scheduling information
- Base and limit register value
- Currently used register
- Changed State
- I/O State information
- Accounting information
Thread
A thread is a flow of execution through the process code, with its own program counter that keeps track of which instruction to execute next, system registers which hold its current working variables, and a stack which contains the execution history.
Each thread belongs to exactly one process and no thread can exist outside a process.
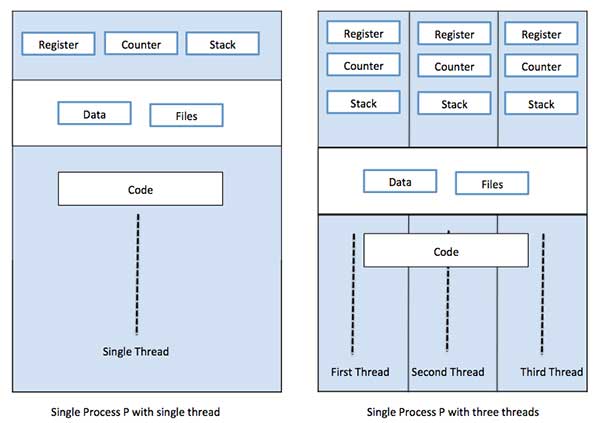
Types of Thread
- User Level Threads − User managed threads.
- Kernel Level Threads − Operating System managed threads acting on kernel, an operating system core.
What is the difference between a process and a thread?
CPU Scheduling: Dispatcher
Another component involved in the CPU scheduling function is the Dispatcher. The dispatcher is the module that gives control of the CPU to the process selected by the short-term scheduler. This function involves:
- Switching context
- Switching to user mode
- Jumping to the proper location in the user program to restart that program from where it left last time.
The dispatcher should be as fast as possible, given that it is invoked during every process switch. The time taken by the dispatcher to stop one process and start another process is known as the Dispatch Latency
Thread Libraries
Thread libraries provide programmers with API for creation and management of threads.
Thread libraries may be implemented either in user space or in kernel space. The user space involves API functions implemented solely within the user space, with no kernel support. The kernel space involves system calls, and requires a kernel with thread library support.
Concurrency
Parallelism
In early timesharing systems, each process was a virtual von Neumann com-
puter; it had a memory space storing both instructions and data, executing in-
structions sequentially according to the semantics of the machine language, and
interacting with the outside world via the operating system through a set of I/O
primitives
They share process-wide resources such as memory and file handles, but
each thread has its own program counter, stack, and local variables.
Threads are sometimes called lightweight processes, and most modern oper-
ating systems treat threads, not processes, as the basic units of scheduling. In
the absence of explicit coordination, threads execute simultaneously and asyn-
chronously with respect to one another.
Since threads share the memory address
space of their owning process, all threads within a process have access to the same
variables and allocate objects from the same heap, which allows finer-grained data
sharing than inter-process mechanisms.
Since the basic unit of scheduling is the thread, a program with only one
thread can run on at most one processor at a time.
Using multiple threads can also help achieve better throughput on single-
processor systems. If a program is single-threaded, the processor remains idle
while it waits for a synchronous I/O operation to complete. In a multithreaded
program, another thread can still run while the first thread is waiting for the I/O
to complete, allowing the application to still make progress during the blocking
I/O.
synchronous I/O
nonblocking I/O
Modern GUI frameworks, such as the AWT and Swing toolkits, replace the
main event loop with an event dispatch thread (EDT).
Annotations documenting thread safety are useful to multiple audiences. If a class is
annotated with @ThreadSafe , users can use it with confidence in a multithreaded
environment, maintainers are put on notice that it makes thread safety guarantees
that must be preserved, and software analysis tools can identify possible coding
errors.
public class UnsafeSequence {
private int value;
/** Returns a unique value. */
public int getNext() {
return value++;
}
}
@ThreadSafe
public class Sequence {
@GuardedBy("this") private int value;
public synchronized int getNext() {
return value++;
}
}
Thread Safety
Informally, an object’s state is its data, stored in state variables such as instance
or static fields.
By shared, we mean that a variable could be accessed by multiple threads;
by mutable, we mean that its value could change during its lifetime.
The primary
mechanism for synchronization in Java is the synchronized keyword, which pro-
vides exclusive locking, but the term “synchronization” also includes the use of
volatile variables, explicit locks, and atomic variables.
A class is thread-safe if it behaves correctly when accessed from multiple
threads, regardless of the scheduling or interleaving of the execution of
those threads by the runtime environment, and with no additional syn-
chronization or other coordination on the part of the calling code.
Stateless objects are always thread-safe.
The possibility of incorrect results in the presence of unlucky timing is so important
in concurrent programming that it has a name: a race condition.
@NotThreadSafe
public class LazyInitRace {
private ExpensiveObject instance = null;
public ExpensiveObject getInstance() {
if (instance == null)
instance = new ExpensiveObject();
return instance;
}
}
LazyInitRace has race conditions that can undermine its correctness. Say that
threads A and B execute getInstance at the same time. A sees that instance
is null , and instantiates a new ExpensiveObject . B also checks if instance is
null . Whether instance is null at this point depends unpredictably on timing,
including the vagaries of scheduling and how long A takes to instantiate the Ex-
pensiveObject and set the instance field. If instance is null when B examines
it, the two callers to getInstance may receive two different results, even though
getInstance is always supposed to return the same instance.
Parallel Workers
In the parallel worker concurrency model a delegator distributes the incoming jobs to different workers. Each worker completes the full job. The workers work in parallel, running in different threads, and possibly on different CPUs.
Critical Section Problem
A Critical Section is a code segment that accesses shared variables and has to be executed as an atomic action. It means that in a group of cooperating processes, at a given point of time, only one process must be executing its critical section
Semaphore
Semaphore is use to manage multiple resources shared between threads and holds a count equivalent to available shared resources.
Binary semaphore is not same as mutex, any thread can release a semaphore, which is not the case in mutex. Semaphore works like signals, and can be used to indicate some event or to maintain the order in which a resource is used or activity is performed.
Mutex
Mutex is the locking mechanism to protect the critical section. It allows multiple threads to access the same shared resource exclusively. Ownership – Thread locking the critical section can only unlock it. Mutex is used when thread does not want any other thread to execute critical section or access critical resource.
Synchronization
Problems of Synchronization
- Bounded Buffer (Producer-Consumer) Problem
- The Readers Writers Problem
- Dining Philosophers Problem
Race Conditions
Race conditions can be avoided by proper thread synchronization in critical sections. Thread synchronization can be achieved using a synchronized block of Java code. Thread synchronization can also be achieved using other synchronization constructs like locks or atomic variables like java.util.concurrent.atomic.AtomicInteger.
Deadlock
Livelock
The nondeterministic nature of the parallel worker model makes it hard to reason about the state of the system at any given point in time. It also makes it harder (if not impossible) to guarantee that one jobs happens before another.
Assembly Line
The workers are organized like workers at an assembly line in a factory. Each worker only performs a part of the full job. When that part is finished the worker forwards the job to the next worker.
What is the difference between concurrency and parallelism?
https://www.quora.com/What-is-the-difference-between-a-process-and-a-thread
https://www.tutorialspoint.com/operating_system/os_processes.html
https://www.studytonight.com/operating-system/operating-system-processes
https://www.studytonight.com/operating-system/
https://en.wikipedia.org/wiki/Context_switch
http://tutorials.jenkov.com/java-concurrency/concurrency-models.html